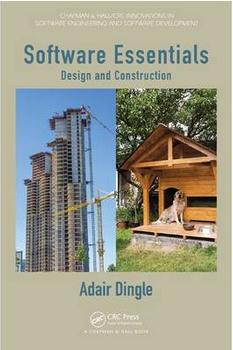
Software Essentials : Design and Construction
[BOOK DESCRIPTION]
About the Coverrequirements are usually minimal. Unlike skyscrapers, doghouses are simple units. They do not require plumbing, electricity, fire alarms, elevators, or ventilation systems, and they do not need to be built to code or pass inspections. The range of complexity in software design is similar. Given available software tools and libraries-many of which are free-hobbyists can build small or short-lived computer apps. Yet, design for software longevity, security, and efficiency can be intricate-as is the design of large-scale systems. How can a software developer prepare to manage such complexity? By understanding the essential building blocks of software design and construction. About the Book: Software Essentials: Design and Construction explicitly defines and illustrates the basic elements of software design and construction, providing a solid understanding of control flow, abstract data types (ADTs), memory, type relationships, and dynamic behavior. This text evaluates the benefits and overhead of object-oriented design (OOD) and analyzes software design options.With a structured but hands-on approach, the book: * Delineates malleable and stable characteristics of software design * Explains how to evaluate the short- and long-term costs and benefits of design decisions * Compares and contrasts design solutions, such as composition versus inheritance * Includes supportive appendices and a glossary of over 200 common terms * Covers key topics such as polymorphism, overloading, and more While extensive examples are given in C# and/or C++, often demonstrating alternative solutions, design-not syntax-remains the focal point of Software Essentials: Design and Construction.
[TABLE OF CONTENTS]
Preface, xi
Acknowledgments, xv
Detailed Book Outline, xvii
Section I Software Construction
Chapter 1 Software Complexity And Modeling 3 (28)
1.1 Modern Software 4 (6)
1.1.1 Software Design 5 (2)
1.1.2 Software Utility 7 (2)
1.1.3 Software Production 9 (1)
1.2 Software Engineering 10 (5)
1.2.1 The Software Development Life Cycle 11 (2)
(SDLC)
1.2.2 Software Process Methodologies 13 (2)
1.3 Models 15 (10)
1.3.1 Requirements Analysis And 19 (2)
Specification
1.3.2 Software Architecture 21 (1)
1.3.3 Model View Controller 22 (1)
1.3.4 Code Construction 23 (2)
1.4 Software Integration 25 (2)
1.5 Documentation 27 (1)
1.6 Summary 28 (1)
Design Insights 29 (2)
Chapter 2 Software Development 31 (28)
2.1 Software Execution 32 (1)
2.2 General Purpose Utility And Support 33 (3)
2.3 Programming Language Evolution 36 (13)
2.3.1 Compilers 36 (6)
2.3.2 Software Design 42 (1)
2.3.3 ADTs 43 (1)
2.3.4 Class Construct 44 (3)
2.3.5 Object-Oriented Programming 47 (2)
Languages
2.4 UML 49 (2)
2.5 Libraries And Frameworks 51 (1)
2.6 Software Construction Fundamentals 52 (2)
2.7 Summary 54 (1)
Design Insights 54 (5)
Section II Software Fundamentals
Chapter 3 Functionality 59 (32)
3.1 Control Flow 60 (14)
3.1.1 Structured Control Flow 62 (6)
3.1.2 Controlled Interruption To 68 (5)
Sequential Execution
3.1.3 Readability 73 (1)
3.2 Boolean Logic 74 (5)
3.2.1 Short-Circuit Evaluation 77 (2)
3.3 Recursion 79 (3)
3.4 Sequential Execution 82 (6)
3.4.1 Optimization 84 (2)
3.4.2 Miming 86 (2)
3.5 Summary 88 (1)
Design Insights 89 (2)
Chapter 4 Memory 91 (28)
4.1 Abstraction Of Memory 92 (2)
4.2 Heap Memory 94 (11)
4.2.1 C++ Deallocation 97 (6)
4.2.2 C#/Java Heap Memory Management 103 (2)
4.3 Memory Overhead 105 (8)
4.3.1 Allocation 106 (1)
4.3.2 Memory Reclamation 107 (2)
4.3.3 Garbage Collection 109 (2)
4.3.4 Reference Counting 111 (2)
4.4 Design: Storage Versus Computation 113 (3)
4.5 Summary 116 (1)
Design Insights 117 (2)
Chapter 5 Design And Documentation 119 (32)
5.1 Object-Oriented Design 120 (1)
5.2 Class Functionality 120 (11)
5.2.1 Constructors 124 (2)
5.2.2 Accessors And Mutators 126 (2)
5.2.3 Utility Functions 128 (2)
5.2.4 Destructors 130 (1)
5.3 Programming By Contract 131 (14)
5.3.1 Defensive Programming 132 (3)
5.3.2 Precondition And Postcondition 135 (2)
5.3.3 Invariants 137 (2)
5.3.4 Design Example 139 (6)
5.3.5 Contractual Expectations 145 (1)
5.4 OO Design Principles 145 (1)
5.5 Summary 146 (1)
Design Insights 147 (4)
Section III Software Design
Chapter 6 Structural Design 151 (30)
6.1 Relationships 152 (5)
6.1.1 Composition 153 (1)
6.1.2 Containment 154 (2)
6.1.3 Class Design: has-a or holds-a? 156 (1)
6.2 Inheritance 157 (10)
6.2.1 Automate Subtype Checking 160 (5)
6.2.2 Inheritance Design 165 (2)
6.3 Code Reuse 167 (10)
6.3.1 Class Design: has-a or is-a? 171 (5)
6.3.2 Contractual Expectations 176 (1)
6.4 OO Design Principles 177 (1)
6.5 Summary 177 (1)
Design Insights 178 (3)
Chapter 7 Behavioral Design 181 (42)
7.1 Inheritance For Functionality 182 (1)
7.2 Polymorphism 182 (4)
7.2.1 Overloading 183 (1)
7.2.2 Generics 184 (1)
7.2.3 Subtype Polymorphism 185 (1)
7.3 Static Binding Versus Dynamic Binding 186 (10)
7.3.1 Heterogeneous Collections 193 (3)
7.4 Virtual Function Table 196 (2)
7.5 Software Design 198 (5)
7.5.1 Abstract Classes 199 (4)
7.6 Real-World Example: Disassembler 203 (8)
7.6.1 Virtual Function Table 208 (3)
7.7 Language Differences 211 (9)
7.7.1 Type Introspection 211 (4)
7.7.2 C++ Virtual Destructors 215 (3)
7.7.3 Accessibility Of C++ Virtual 218 (2)
Functions
7.8 OO Design Principles 220 (1)
7.9 Summary 220 (1)
Design Insights 220 (3)
Chapter 8 Design Alternatives And Perspectives 223 (46)
8.1 Comparative Design 224 (1)
8.2 Class Design Types 224 (12)
8.2.1 Concrete Class 224 (2)
8.2.2 Abstract Class 226 (2)
8.2.3 Node Class 228 (1)
8.2.4 Wrappers 229 (2)
8.2.5 Delegate 231 (2)
8.2.6 Handle: Smart Pointers 233 (3)
8.3 Design Specifications For Inheritance 236 (3)
8.4 Inheritance Versus Composition 239 (5)
8.5 Multiple Inheritance 244 (11)
8.5.1 Multiple Inheritance Imperfections 246 (5)
8.5.2 Single Inheritance With Composition 251 (2)
8.5.3 Simulated Design Without Inheritance 253 (2)
8.6 Multiple Inheritance Design 255 (7)
8.6.1 Evaluating Design Options 260 (1)
8.6.2 Relevance Of Type 261 (1)
8.7 OO Design Principles 262 (1)
8.8 Summary 263 (1)
Design Insights 264 (5)
Section IV Software Durability
Chapter 9 Software Correctness 269 (16)
9.1 Exceptions 269 (6)
9.1.1 Exceptions And Software Design 274 (1)
9.2 Testing Design 275 (6)
9.2.1 Scale 275 (2)
9.2.2 Perspective 277 (1)
9.2.3 Coverage 278 (1)
9.2.4 Data Values 279 (2)
9.3 Software Qualities 281 (2)
9.4 Summary 283 (1)
Design Insights 283 (2)
Chapter 10 Software Longevity 285 (20)
10.1 Software Maintenance 286 (1)
10.2 Software Evolution 286 (3)
10.3 Nonfunctional Properties 289 (6)
10.4 Refactoring 295 (5)
10.5 Reverse Engineering 300 (3)
10.6 Summary 303 (1)
Design Insights 303 (2)
Glossary: Definitions And Conceptual Details, 305 (24)
References, 329 (2)
Appendix A: Memory And The Pointer Construct, 331 (16)
Appendix B: Heap Memory And Aliases, 347 (22)
Appendix C: Function Pointers, 369 (10)
Appendix D: Operator Overloading, 379 (28)
Index, 407