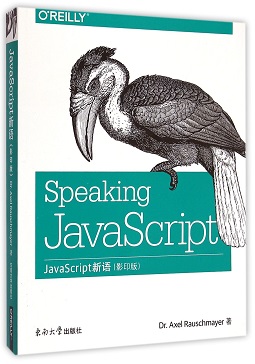
Speaking JavaScript
[Book Description]
Like it or not, JavaScript is everywhere these days—from browser to server to mobile—and now you, too, need to learn the language or dive deeper than you have. This concise book guides you into and through JavaScript, written by a veteran programmer who once found himself in the same position.
Speaking JavaScript helps you approach the language with four standalone sections. First, a quick-start guide teaches you just enough of the language to help you be productive right away. More experienced JavaScript programmers will find a complete and easy-to-read reference that covers each language feature in depth. Complete contents include:
JavaScript quick start: Familiar with object-oriented programming? This part helps you learn JavaScript quickly and properly.
JavaScript in depth: Learn details of ECMAScript 5, from syntax, variables, functions, and object-oriented programming to regular expressions and JSON with lots of examples. Pick a topic and jump in.
Background: Understand JavaScript’s history and its relationship with other programming languages.
Tips, tools, and libraries: Survey existing style guides, best practices, advanced techniques, module systems, package managers, build tools, and learning resources.
[Table of Contents]
Preface
Part Ⅰ. JavaScript Quick Start
1. Basic JavaScript
Background
Syntax
Variables and Assignment
Values
Booleans
Numbers
Operators
Strings
Statements
Functions
Exception Handling
Strict Mode
Variable Scoping and Closures
Objects and Constructors
Arrays
Regular Expressions
Math
Other Functionality of the Standard Library
Part Ⅱ. Background
2. Why JavaScript?
Is JavaScript Freely Available?
Is JavaScript Elegant?
Is JavaScript Useful?
Does JavaScript Have Good Tools?
Is JavaScript Fast Enough?
Is JavaScript Widely Used?
Does JavaScript Have a Future?
Conclusion
3. The Nature oflavaScript
Quirks and Unorthodox Features
Elegant Parts
Influences
4. How JavaScript Was Created
5. Standardization: ECMAScript
6. HistoricaIJavaScript Milestones
PartⅢ. JavaScriptin Depth
7. JavaScript's Syntax
An Overview of the Syntax
Comments
Expressions Versus Statements
Control Flow Statements and Blocks
Rules for Using Semicolons
Legal Identifiers
Invoking Methods on Number Literals
Strict Mode
8. Values
JavaScript's Type System
Primitive Values Versus Objects
Primitive Values
Objects
undefined and null
Wrapper Objects for Primitives
Type Coercion
9. Operators
Operators and Objects
Assignment Operators
Equality Operators: === Versus ==
Ordering Operators
The Plus Operator (+)
Operators for Booleans and Numbers
Spe Operators
Categorizing Values via typeof and instanceof
Object Operators
10. Booleans
Converting to Boolean
Logical Operators
Equality Operators, Ordering Operators
The Function Boolean
11. Numbers
Number Literals
Converting to Number
Spe Number Values
The Internal Representation of Numbers
Handling Rounding Errors
Integers in JavaScript
Converting to Integer
Arithmetic Operators
Bitwise Operators
The Function Number
Number Constructor Properties
Number Prototype Methods
Functions for Numbers
Sources for This Chapter
12. Strings
String Literals
Escaping in String Literals
Character Access
Converting to String
Comparing Strings
Concatenating Strings
The Function String
String Constructor Method
String Instance Property length
String Prototype Methods
13. Statements
Declaring and Assigning Variables
The Bodies of Loops and Conditionals
Loops
Conditionals
The with Statement
The debugger Statement
14. Exception Handling
What Is Exception Handling?
Exception Handling in ]avaScript
Error Constructors
Stack Traces
Implementing Your Own Error Constructor
15. Functions
The Three Roles of Functions in JavaScript
Terminology: "Parameter" Versus "Argument"
Defining Functions
Hoisting
The Name of a Function
Which Is Better: A Function Declaration or a Function Expression?
More Control over Function Calls: call(), apply(), and bind()
Handling Missing or Extra Parameters
Named Parameters
16. Variables: Scopes, Environments, and Closures
Declaring a Variable
Background: Static Versus Dynamic
Background: The&n
......